Ready to take a spin on probability with Python? Let’s build a Monte Carlo simulation for a roulette wheel and visualize the possible outcomes as random walks. Buckle up!
1. Define the Model:
A roulette wheel has 37 slots (European version): 18 red, 18 black, and 1 green (0). Your goal is to simulate placing bets on red and track your winnings after multiple spins.
2. Import Libraries:
import random
import matplotlib.pyplot as plt
3. Set Up Spin Function:
def spin_roulette():
"""Simulates a single roulette spin and returns the winning color (red or green)."""
result = random.randint(0, 36)
if result in range(18):
return "red"
elif result in range(18, 36):
return "black"
else:
return "green"
4. Implement the Simulation:
num_spins = 1000 # Number of spins to simulate
initial_amount = 100 # Starting amount
current_amount = initial_amount
winning_history = [] # Store winnings/losses for each spin
for i in range(num_spins):
# Place a bet on red
current_amount -= 1
# Spin the wheel
result = spin_roulette()
# Check if it lands on red (win)
if result == "red":
current_amount += 2 # Gain double the bet amount
# Store the change in amount for this spin
winning_history.append(current_amount - initial_amount)
# Calculate final amount and winning percentage
final_amount = current_amount
win_rate = (final_amount - initial_amount) / initial_amount * 100
5. Visualize Random Walks:
# Plot the winning history as a line graph
plt.plot(winning_history)
plt.xlabel("Spin Number")
plt.ylabel("Amount (Relative to Initial)")
plt.title("Monte Carlo Simulation: Roulette Winnings (Red Bets)")
plt.grid(True)
plt.show()
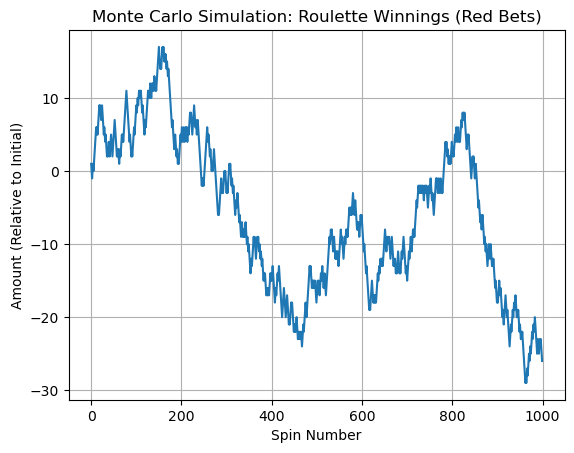
6. Running Multiple Simulations
import random
import matplotlib.pyplot as plt
def spin_roulette():
# Simulates a single roulette spin and returns the winning color (red or green).
result = random.randint(0, 36)
if result in range(18):
return "red"
elif result in range(18, 36):
return "black"
else:
return "green"
def run_simulation(num_spins, initial_amount, num_sims):
# Runs multiple simulations and returns a list of final amounts.
winning_histories = []
for _ in range(num_sims):
current_amount = initial_amount
winning_history = []
for i in range(num_spins):
current_amount -= 1
result = spin_roulette()
if result == "red":
current_amount += 2
winning_history.append(current_amount - initial_amount)
winning_histories.append(winning_history)
return winning_histories
# Set parameters
num_spins = 1000
initial_amount = 100
num_sims = 30 # Number of simulations to run
# Run simulations and get winning histories
winning_histories = run_simulation(num_spins, initial_amount, num_sims)
# Set transparency for clearer visualization
transparency = 0.3
# Plot each simulation's random walk
for history in winning_histories:
plt.plot(history, alpha=transparency)
# Configure and show the plot
plt.xlabel("Spin Number")
plt.ylabel("Amount (Relative to Initial)")
plt.title("Monte Carlo Simulation: 10 Roulette Winnings (Red Bets)")
plt.grid(True)
plt.legend() # Add legend to show individual simulations
plt.show()
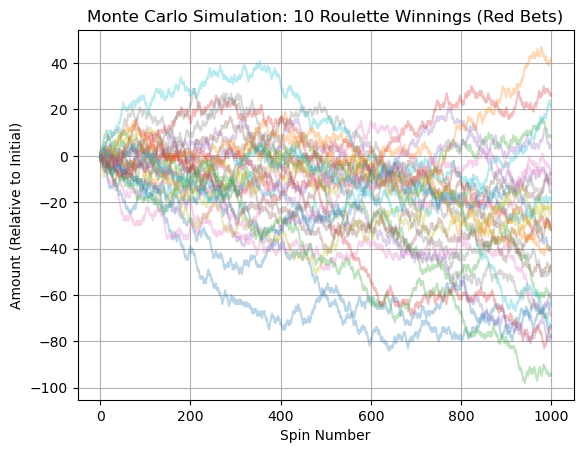
This code:
- Defines a
run_simulation
function that takes the number of spins, initial amount, and number of simulations as input and returns a list of winning histories for each simulation. - Sets parameters for the simulation.
- Runs the simulations and stores the winning histories.
- Plots each simulation’s random walk with a slight transparency to avoid overlapping lines.
7. Bonus: Analyze Results:
- Calculate the average win/loss per spin.
- Try different bet strategies and compare their outcomes.
- Explore more complex visualizations like histograms or boxplots.
Leave a Reply